Ecosyste.ms: Repos
An open API service providing repository metadata for many open source software ecosystems.
Package Usage: go: github.com/gocql/gocql
Package gocql implements a fast and robust Cassandra driver for the
Go programming language.
Pass a list of initial node IP addresses to NewCluster to create a new cluster configuration:
Port can be specified as part of the address, the above is equivalent to:
It is recommended to use the value set in the Cassandra config for broadcast_address or listen_address,
an IP address not a domain name. This is because events from Cassandra will use the configured IP
address, which is used to index connected hosts. If the domain name specified resolves to more than 1 IP address
then the driver may connect multiple times to the same host, and will not mark the node being down or up from events.
Then you can customize more options (see ClusterConfig):
The driver tries to automatically detect the protocol version to use if not set, but you might want to set the
protocol version explicitly, as it's not defined which version will be used in certain situations (for example
during upgrade of the cluster when some of the nodes support different set of protocol versions than other nodes).
The driver advertises the module name and version in the STARTUP message, so servers are able to detect the version.
If you use replace directive in go.mod, the driver will send information about the replacement module instead.
When ready, create a session from the configuration. Don't forget to Close the session once you are done with it:
CQL protocol uses a SASL-based authentication mechanism and so consists of an exchange of server challenges and
client response pairs. The details of the exchanged messages depend on the authenticator used.
To use authentication, set ClusterConfig.Authenticator or ClusterConfig.AuthProvider.
PasswordAuthenticator is provided to use for username/password authentication:
It is possible to secure traffic between the client and server with TLS.
To use TLS, set the ClusterConfig.SslOpts field. SslOptions embeds *tls.Config so you can set that directly.
There are also helpers to load keys/certificates from files.
Warning: Due to historical reasons, the SslOptions is insecure by default, so you need to set EnableHostVerification
to true if no Config is set. Most users should set SslOptions.Config to a *tls.Config.
SslOptions and Config.InsecureSkipVerify interact as follows:
For example:
To route queries to local DC first, use DCAwareRoundRobinPolicy. For example, if the datacenter you
want to primarily connect is called dc1 (as configured in the database):
The driver can route queries to nodes that hold data replicas based on partition key (preferring local DC).
Note that TokenAwareHostPolicy can take options such as gocql.ShuffleReplicas and gocql.NonLocalReplicasFallback.
We recommend running with a token aware host policy in production for maximum performance.
The driver can only use token-aware routing for queries where all partition key columns are query parameters.
For example, instead of
use
The DCAwareRoundRobinPolicy can be replaced with RackAwareRoundRobinPolicy, which takes two parameters, datacenter and rack.
Instead of dividing hosts with two tiers (local datacenter and remote datacenters) it divides hosts into three
(the local rack, the rest of the local datacenter, and everything else).
RackAwareRoundRobinPolicy can be combined with TokenAwareHostPolicy in the same way as DCAwareRoundRobinPolicy.
Create queries with Session.Query. Query values must not be reused between different executions and must not be
modified after starting execution of the query.
To execute a query without reading results, use Query.Exec:
Single row can be read by calling Query.Scan:
Multiple rows can be read using Iter.Scanner:
See Example for complete example.
The driver automatically prepares DML queries (SELECT/INSERT/UPDATE/DELETE/BATCH statements) and maintains a cache
of prepared statements.
CQL protocol does not support preparing other query types.
When using CQL protocol >= 4, it is possible to use gocql.UnsetValue as the bound value of a column.
This will cause the database to ignore writing the column.
The main advantage is the ability to keep the same prepared statement even when you don't
want to update some fields, where before you needed to make another prepared statement.
Session is safe to use from multiple goroutines, so to execute multiple concurrent queries, just execute them
from several worker goroutines. Gocql provides synchronously-looking API (as recommended for Go APIs) and the queries
are executed asynchronously at the protocol level.
Null values are are unmarshalled as zero value of the type. If you need to distinguish for example between text
column being null and empty string, you can unmarshal into *string variable instead of string.
See Example_nulls for full example.
The driver reuses backing memory of slices when unmarshalling. This is an optimization so that a buffer does not
need to be allocated for every processed row. However, you need to be careful when storing the slices to other
memory structures.
When you want to save the data for later use, pass a new slice every time. A common pattern is to declare the
slice variable within the scanner loop:
The driver supports paging of results with automatic prefetch, see ClusterConfig.PageSize, Session.SetPrefetch,
Query.PageSize, and Query.Prefetch.
It is also possible to control the paging manually with Query.PageState (this disables automatic prefetch).
Manual paging is useful if you want to store the page state externally, for example in a URL to allow users
browse pages in a result. You might want to sign/encrypt the paging state when exposing it externally since
it contains data from primary keys.
Paging state is specific to the CQL protocol version and the exact query used. It is meant as opaque state that
should not be modified. If you send paging state from different query or protocol version, then the behaviour
is not defined (you might get unexpected results or an error from the server). For example, do not send paging state
returned by node using protocol version 3 to a node using protocol version 4. Also, when using protocol version 4,
paging state between Cassandra 2.2 and 3.0 is incompatible (https://issues.apache.org/jira/browse/CASSANDRA-10880).
The driver does not check whether the paging state is from the same protocol version/statement.
You might want to validate yourself as this could be a problem if you store paging state externally.
For example, if you store paging state in a URL, the URLs might become broken when you upgrade your cluster.
Call Query.PageState(nil) to fetch just the first page of the query results. Pass the page state returned by
Iter.PageState to Query.PageState of a subsequent query to get the next page. If the length of slice returned
by Iter.PageState is zero, there are no more pages available (or an error occurred).
Using too low values of PageSize will negatively affect performance, a value below 100 is probably too low.
While Cassandra returns exactly PageSize items (except for last page) in a page currently, the protocol authors
explicitly reserved the right to return smaller or larger amount of items in a page for performance reasons, so don't
rely on the page having the exact count of items.
See Example_paging for an example of manual paging.
There are certain situations when you don't know the list of columns in advance, mainly when the query is supplied
by the user. Iter.Columns, Iter.RowData, Iter.MapScan and Iter.SliceMap can be used to handle this case.
See Example_dynamicColumns.
The CQL protocol supports sending batches of DML statements (INSERT/UPDATE/DELETE) and so does gocql.
Use Session.NewBatch to create a new batch and then fill-in details of individual queries.
Then execute the batch with Session.ExecuteBatch.
Logged batches ensure atomicity, either all or none of the operations in the batch will succeed, but they have
overhead to ensure this property.
Unlogged batches don't have the overhead of logged batches, but don't guarantee atomicity.
Updates of counters are handled specially by Cassandra so batches of counter updates have to use CounterBatch type.
A counter batch can only contain statements to update counters.
For unlogged batches it is recommended to send only single-partition batches (i.e. all statements in the batch should
involve only a single partition).
Multi-partition batch needs to be split by the coordinator node and re-sent to
correct nodes.
With single-partition batches you can send the batch directly to the node for the partition without incurring the
additional network hop.
It is also possible to pass entire BEGIN BATCH .. APPLY BATCH statement to Query.Exec.
There are differences how those are executed.
BEGIN BATCH statement passed to Query.Exec is prepared as a whole in a single statement.
Session.ExecuteBatch prepares individual statements in the batch.
If you have variable-length batches using the same statement, using Session.ExecuteBatch is more efficient.
See Example_batch for an example.
Query.ScanCAS or Query.MapScanCAS can be used to execute a single-statement lightweight transaction (an
INSERT/UPDATE .. IF statement) and reading its result. See example for Query.MapScanCAS.
Multiple-statement lightweight transactions can be executed as a logged batch that contains at least one conditional
statement. All the conditions must return true for the batch to be applied. You can use Session.ExecuteBatchCAS and
Session.MapExecuteBatchCAS when executing the batch to learn about the result of the LWT. See example for
Session.MapExecuteBatchCAS.
Queries can be marked as idempotent. Marking the query as idempotent tells the driver that the query can be executed
multiple times without affecting its result. Non-idempotent queries are not eligible for retrying nor speculative
execution.
Idempotent queries are retried in case of errors based on the configured RetryPolicy.
Queries can be retried even before they fail by setting a SpeculativeExecutionPolicy. The policy can
cause the driver to retry on a different node if the query is taking longer than a specified delay even before the
driver receives an error or timeout from the server. When a query is speculatively executed, the original execution
is still executing. The two parallel executions of the query race to return a result, the first received result will
be returned.
UDTs can be mapped (un)marshaled from/to map[string]interface{} a Go struct (or a type implementing
UDTUnmarshaler, UDTMarshaler, Unmarshaler or Marshaler interfaces).
For structs, cql tag can be used to specify the CQL field name to be mapped to a struct field:
See Example_userDefinedTypesMap, Example_userDefinedTypesStruct, ExampleUDTMarshaler, ExampleUDTUnmarshaler.
It is possible to provide observer implementations that could be used to gather metrics:
CQL protocol also supports tracing of queries. When enabled, the database will write information about
internal events that happened during execution of the query. You can use Query.Trace to request tracing and receive
the session ID that the database used to store the trace information in system_traces.sessions and
system_traces.events tables. NewTraceWriter returns an implementation of Tracer that writes the events to a writer.
Gathering trace information might be essential for debugging and optimizing queries, but writing traces has overhead,
so this feature should not be used on production systems with very high load unless you know what you are doing.
Example_batch demonstrates how to execute a batch of statements.
Example_dynamicColumns demonstrates how to handle dynamic column list.
Example_marshalerUnmarshaler demonstrates how to implement a Marshaler and Unmarshaler.
Example_nulls demonstrates how to distinguish between null and zero value when needed.
Null values are unmarshalled as zero value of the type. If you need to distinguish for example between text
column being null and empty string, you can unmarshal into *string field.
Example_paging demonstrates how to manually fetch pages and use page state.
See also package documentation about paging.
Example_set demonstrates how to use sets.
Example_userDefinedTypesMap demonstrates how to work with user-defined types as maps.
See also Example_userDefinedTypesStruct and examples for UDTMarshaler and UDTUnmarshaler if you want to map to structs.
Example_userDefinedTypesStruct demonstrates how to work with user-defined types as structs.
See also examples for UDTMarshaler and UDTUnmarshaler if you need more control/better performance.
12 versions
Latest release: 8 months ago
5,320 dependent packages
View more package details: https://packages.ecosyste.ms/registries/proxy.golang.org/packages/github.com/gocql/gocql
View more repository details: https://repos.ecosyste.ms/hosts/GitHub/repositories/gocql%2Fgocql
Dependent Repos 8,661
spolti/kie-cloud-operator Fork of kiegroup/kie-cloud-operator
OCP Operator for KIE- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 100 MB - Last synced: 8 months ago - Pushed: 8 months ago
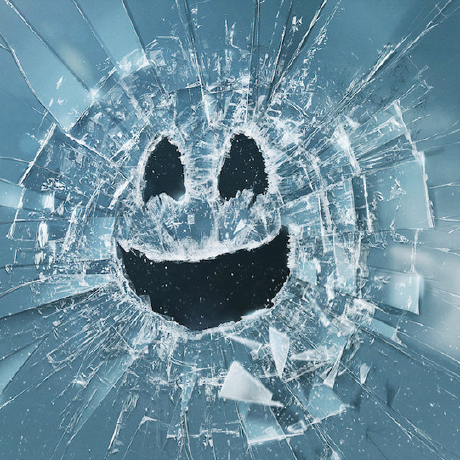
tharun208/loki Fork of grafana/loki
Like Prometheus, but for logs.- v0.0.0-20200526081602-cd04bd7f22a7 go.mod
Size: 168 MB - Last synced: 17 days ago - Pushed: about 1 year ago

jan--f/telemeter Fork of openshift/telemeter
Prometheus push federation- v0.0.0-20190301043612-f6df8288f9b4 tools/go.sum
- v0.0.0-20200121121104-95d072f1b5bb tools/go.sum
Size: 35 MB - Last synced: 11 months ago - Pushed: 11 months ago

carlkyrillos/integreatly-operator Fork of integr8ly/integreatly-operator
An Openshift Operator based on the Operator SDK for installing and reconciling Integreatly services- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 126 MB - Last synced: 16 days ago - Pushed: 16 days ago

jrivera-px/operator Fork of libopenstorage/operator
Storage operator for Kubernetes- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20200121121104-95d072f1b5bb go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 go.sum
Size: 34.9 MB - Last synced: about 1 year ago - Pushed: about 1 year ago
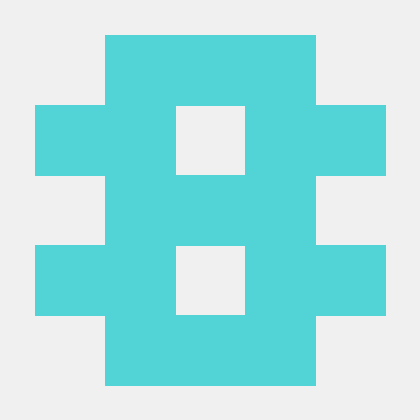
slachiewicz/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShift- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1alpha1/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 kubernetes-model-generator/go.sum
Size: 466 MB - Last synced: about 5 hours ago - Pushed: 1 day ago

cilium/cilium-olm 📦
- v0.0.0-20190301043612-f6df8288f9b4 tools/go.sum
Size: 982 KB - Last synced: 9 months ago - Pushed: 12 months ago
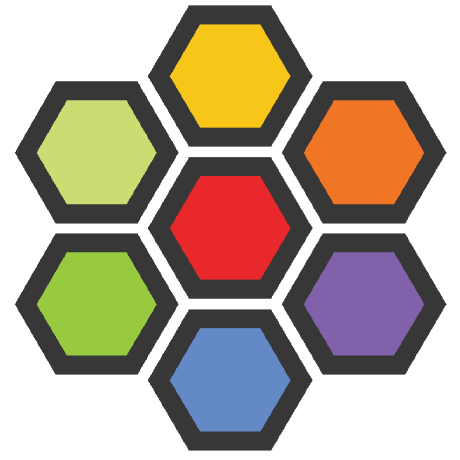
Boroda76/go_service_naked
- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 791 KB - Last synced: 16 days ago - Pushed: about 1 year ago
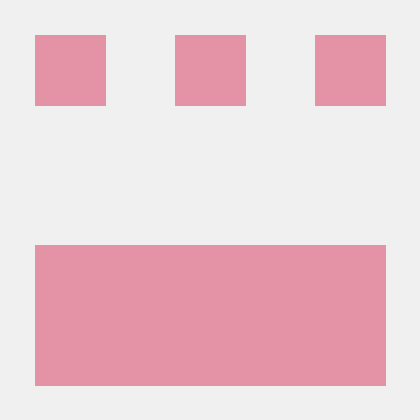
pulumi/pulumi-kubernetes-operator
A Kubernetes Operator that automates the deployment of Pulumi Stacks- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 1.47 MB - Last synced: 2 days ago - Pushed: 2 days ago

moov-io/irs
Internal Revenue Service (IRS) Filing Information Returns Electronically (FIRE)- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 1.4 MB - Last synced: about 3 hours ago - Pushed: about 4 hours ago

moov-io/ach-test-harness
Programmatic and configurable ACH scenario testing of returns, NOC/corrections, reconciliation, etc.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 950 KB - Last synced: 2 days ago - Pushed: 3 days ago

VibrentHealth/keycloak-operator Fork of keycloak/keycloak-operator
A Kubernetes Operator based on the Operator SDK for syncing resources in Keycloak- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 12.4 MB - Last synced: 2 months ago - Pushed: 2 months ago

sknot-rh/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShift- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1alpha1/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 kubernetes-model-generator/go.sum
Size: 442 MB - Last synced: about 1 year ago - Pushed: over 1 year ago
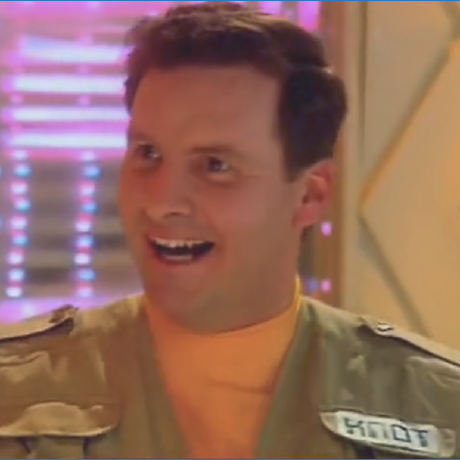
tts-tblock/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShift- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1alpha1/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 extensions/open-cluster-management/generator-agent/go.sum
- v0.0.0-20190402132108-0e1d5de854df extensions/open-cluster-management/generator-agent/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 extensions/open-cluster-management/generator-observability/go.sum
- v0.0.0-20190402132108-0e1d5de854df extensions/open-cluster-management/generator-observability/go.sum
- v0.0.0-20200121121104-95d072f1b5bb extensions/open-cluster-management/generator-observability/go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 extensions/open-cluster-management/generator-observability/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 extensions/open-cluster-management/generator-operator/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 kubernetes-model-generator/go.sum
Size: 449 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

raystack/compass
Compass is an enterprise data catalog that makes it easy to find, understand, and govern data.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 4.69 MB - Last synced: 7 months ago - Pushed: 7 months ago

trustbloc/auth 📦
Authorization Server- v0.0.0-20200624222514-34081eda590e test/bdd/mock/loginconsent/go.sum
Size: 2.24 MB - Last synced: about 2 months ago - Pushed: about 1 year ago

erda-project/kubeprober
Large-scale Kubernetes cluster diagnostic tool.- v0.0.0-20200131111108-92af2e088537 go.sum
- v0.0.0-20210707082121-9a3953d1826d go.sum
Size: 268 MB - Last synced: 18 days ago - Pushed: 4 months ago
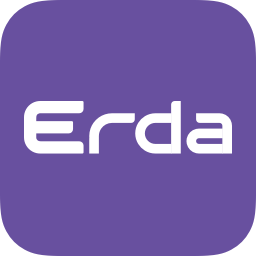
kokizzu/pirsch Fork of pirsch-analytics/pirsch
Pirsch is a drop-in, server-side, no-cookie, and privacy-focused analytics solution for Go.- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 2.11 MB - Last synced: 19 days ago - Pushed: 19 days ago

kubesphere/notification-manager
K8s native notification management with multi-tenancy support- v0.0.0-20190301043612-f6df8288f9b4 sidecar/kubesphere/3.1.0/go.sum
- v0.0.0-20200121121104-95d072f1b5bb sidecar/kubesphere/3.1.0/go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 sidecar/kubesphere/3.1.0/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 sidecar/kubesphere/3.2.0/go.sum
- v0.0.0-20200121121104-95d072f1b5bb sidecar/kubesphere/3.2.0/go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 sidecar/kubesphere/3.2.0/go.sum
Size: 2.23 MB - Last synced: 21 days ago - Pushed: about 1 month ago
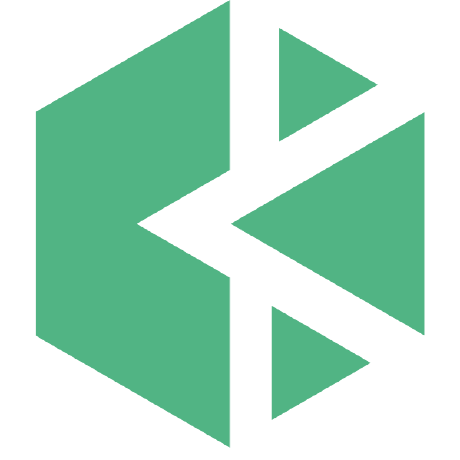
boostchicken/opentelemetry-collector-contrib Fork of open-telemetry/opentelemetry-collector-contrib
Contrib repository for the OpenTelemetry Collector- v0.0.0-20200228163523-cd4b606dd2fb cmd/configschema/go.sum
- v0.0.0-20211222173705-d73e6b1002a7 cmd/configschema/go.sum
- v0.0.0-20200228163523-cd4b606dd2fb cmd/otelcontribcol/go.sum
- v0.0.0-20211222173705-d73e6b1002a7 cmd/otelcontribcol/go.sum
- v0.0.0-20200228163523-cd4b606dd2fb cmd/oteltestbedcol/go.sum
- v0.0.0-20211222173705-d73e6b1002a7 cmd/oteltestbedcol/go.sum
- v0.0.0-20200228163523-cd4b606dd2fb exporter/signalfxexporter/go.sum
- v0.0.0-20211222173705-d73e6b1002a7 exporter/signalfxexporter/go.sum
- v0.0.0-20200228163523-cd4b606dd2fb go.sum
- v0.0.0-20211222173705-d73e6b1002a7 go.sum
Size: 262 MB - Last synced: 3 days ago - Pushed: 5 months ago
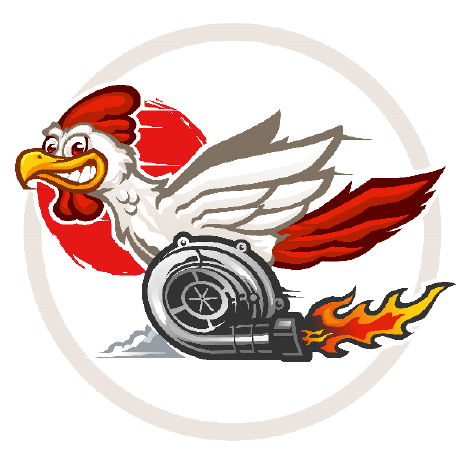
moov-io/base
core libraries used in Moov projects- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 1.48 MB - Last synced: 1 day ago - Pushed: 1 day ago

SadmiB/seaweedfs Fork of seaweedfs/seaweedfs
SeaweedFS is a fast distributed storage system for blobs, objects, files, and data lake, for billions of files! Blob store has O(1) disk seek, cloud tiering. Filer supports Cloud Drive, cross-DC active-active replication, Kubernetes, POSIX FUSE mount, S3 API, S3 Gateway, Hadoop, WebDAV, encryption, Erasure Coding.- v0.0.0-20210707082121-9a3953d1826d go.mod
- v0.0.0-20210707082121-9a3953d1826d go.sum
Size: 40.6 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

me-diru/parca Fork of parca-dev/parca
Continuous profiling for analysis of CPU, memory usage over time, and down to the line number. Saving infrastructure cost, improving performance, and increasing reliability.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20200121121104-95d072f1b5bb go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 go.sum
Size: 13.1 MB - Last synced: 3 days ago - Pushed: about 1 year ago

buildpacks/registry-api
API for searching and reading the Buildpack Registry- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 1.79 MB - Last synced: 19 days ago - Pushed: 7 months ago
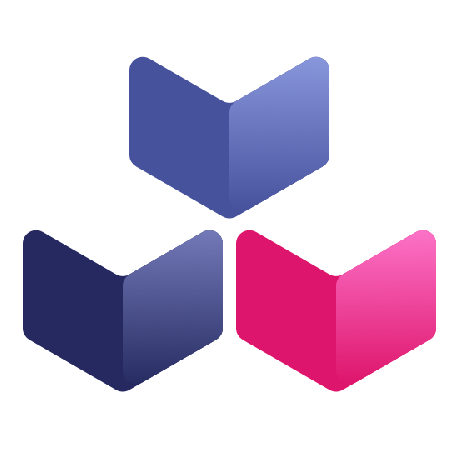
blrm/osde2e Fork of openshift/osde2e
Repository of e2e test plumbing for OpenShift Dedicated- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 431 MB - Last synced: 12 months ago - Pushed: about 1 year ago
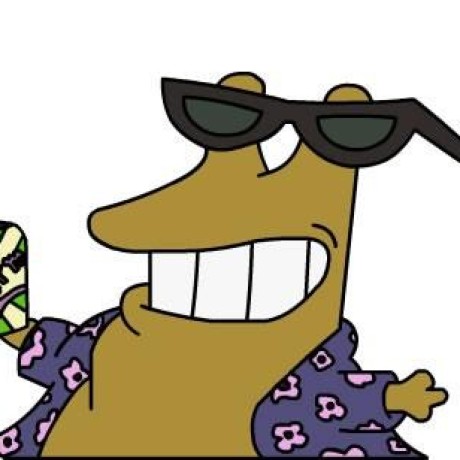
aswinsuryan/submariner-operator Fork of submariner-io/submariner-operator
The submariner-operator installs and maintains your submariner deployment.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 5.53 MB - Last synced: 9 days ago - Pushed: 9 days ago

jeschkies/loki Fork of grafana/loki
Like Prometheus, but for logs.- v0.0.0-20200526081602-cd04bd7f22a7 go.mod
Size: 199 MB - Last synced: 17 days ago - Pushed: 19 days ago
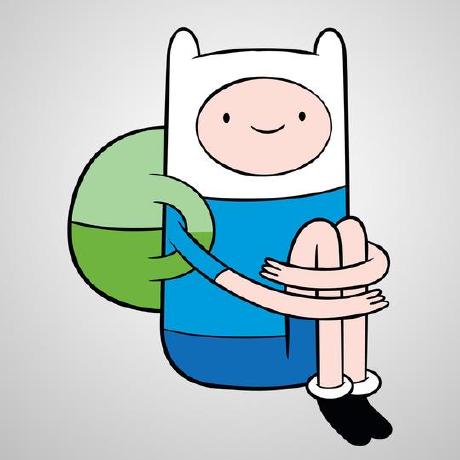
alrs/magma Fork of magma/magma
Platform for building access networks and modular network services- v0.0.0-20190301043612-f6df8288f9b4 cwf/k8s/cwf_operator/go.sum
Size: 199 MB - Last synced: about 1 year ago - Pushed: over 1 year ago

sanekkurt/telegraf Fork of influxdata/telegraf
The plugin-driven server agent for collecting & reporting metrics.- v0.0.0-20200228163523-cd4b606dd2fb go.sum
Size: 43.5 MB - Last synced: about 1 year ago - Pushed: almost 2 years ago

getsynq/migrate Fork of golang-migrate/migrate
Database migrations. CLI and Golang library.- v0.0.0-20210515062232-b7ef815b4556 go.mod
- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 1.63 MB - Last synced: 10 months ago - Pushed: almost 2 years ago

tpantelis/submariner-addon Fork of stolostron/submariner-addon
An addon of submariner in ocm to provide network connectivity and service discovery among clusters.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 35.1 MB - Last synced: 2 days ago - Pushed: 3 days ago

Tessg22/osde2e Fork of openshift/osde2e
Repository of e2e test plumbing for OpenShift Dedicated- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 465 MB - Last synced: about 1 year ago - Pushed: about 1 year ago
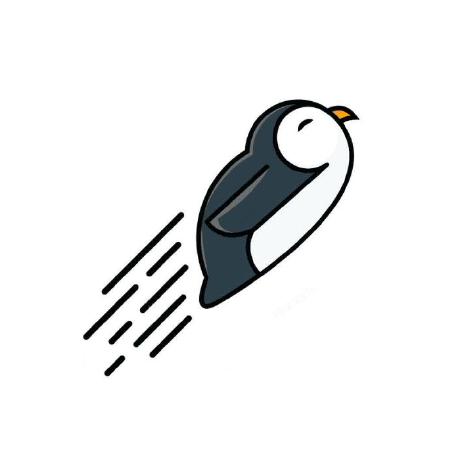
monicawoj/parca Fork of parca-dev/parca
Continuous profiling for analysis of CPU, memory usage over time, and down to the line number. Saving infrastructure cost, improving performance, and increasing reliability.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20200121121104-95d072f1b5bb go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 go.sum
Size: 26.5 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

moov-io/go-sftp
A Go client for performing common SFTP operations- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 753 KB - Last synced: 26 days ago - Pushed: 26 days ago

xp-1000/signalfx-agent Fork of signalfx/signalfx-agent
The SignalFx Smart Agent- v0.0.0-20200228163523-cd4b606dd2fb go.sum
- v0.0.0-20210401103645-80ab1e13e309 go.sum
- v0.0.0-20200228163523-cd4b606dd2fb pkg/apm/go.sum
Size: 36.4 MB - Last synced: 9 months ago - Pushed: about 1 year ago
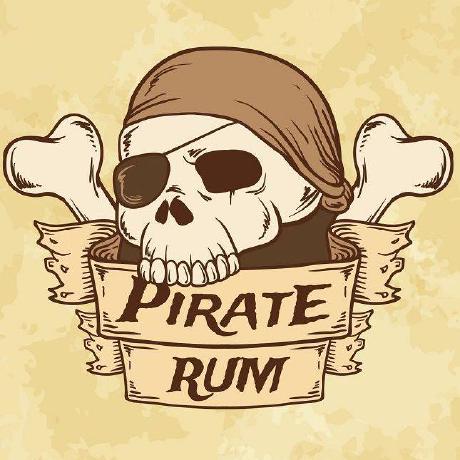
romm80/shortener
- v0.0.0-20210515062232-b7ef815b4556 go.sum
Size: 7.01 MB - Last synced: about 1 year ago - Pushed: almost 2 years ago

topfreegames/maestro
Maestro: Game Room Scheduler- v0.0.0-20190301043612-f6df8288f9b4 e2e/go.sum
- v0.0.0-20190301043612-f6df8288f9b4 go.mod
- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 10.8 MB - Last synced: 2 days ago - Pushed: 2 days ago
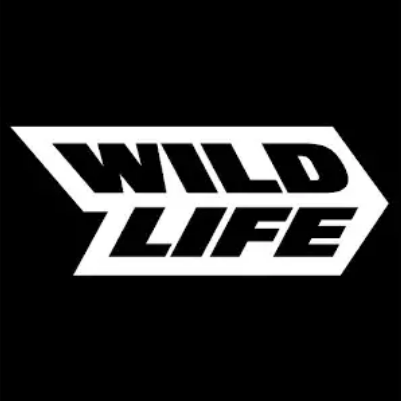
HadesArchitect/GrafanaCassandraDatasource
Apache Cassandra Datasource for Grafana- v0.0.0-20201215165327-e49edf966d90 backend/go.mod
- v0.0.0-20201215165327-e49edf966d90 backend/go.sum
Size: 2.1 MB - Last synced: 7 months ago - Pushed: 7 months ago
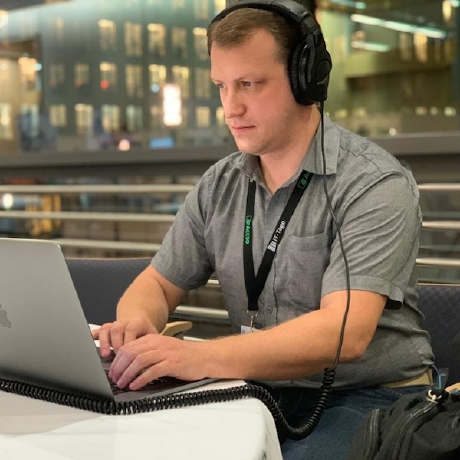
xl3lackout/Erupe
This is a community upload of a community project. The amount of people who worked on it is innumerous, and hard to keep track of. But id like to contribute this to the efforts of Ando, Fists Team, the French Team, Mai's Team and the many wondeful members of the MHFZ community who gave their time and energy to help us. No matter the relations, these files will remain public and open source, free for all to use and modify.- v0.0.0-20211015133455-b225f9b53fa1 Erupe/go.mod
- v0.0.0-20210515062232-b7ef815b4556 Erupe/go.sum
- v0.0.0-20211015133455-b225f9b53fa1 Erupe/go.sum
Size: 666 MB - Last synced: 3 days ago - Pushed: over 1 year ago

TMFRook/modeldb Fork of VertaAI/modeldb
Open Source ML Model Versioning, Metadata, and Experiment Management- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 35 MB - Last synced: 20 days ago - Pushed: almost 2 years ago

SmilyOrg/photofield
Experimental fast photo viewer.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 12.6 MB - Last synced: 2 months ago - Pushed: 2 months ago

dgfug/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20200121121104-95d072f1b5bb go.sum
- v0.0.0-20200228163523-cd4b606dd2fb go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 go.sum
Size: 420 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

boost-entropy-k8s/k8ssandra Fork of k8ssandra/k8ssandra
K8ssandra is an open-source distribution of Apache Cassandra for Kubernetes including API services and operational tooling.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
Size: 25 MB - Last synced: 21 days ago - Pushed: about 1 month ago
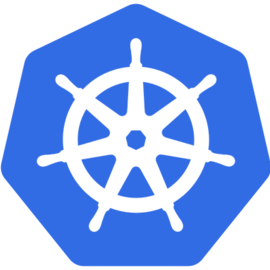
coopstah13/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShift- v0.0.0-20190301043612-f6df8288f9b4 extensions/camel-k/generator-v1/go.sum
Size: 446 MB - Last synced: 9 months ago - Pushed: about 1 year ago

ekmixon/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20200121121104-95d072f1b5bb go.sum
- v0.0.0-20200228163523-cd4b606dd2fb go.sum
- v0.0.0-20200526081602-cd04bd7f22a7 go.sum
Size: 462 MB - Last synced: 9 days ago - Pushed: 9 days ago
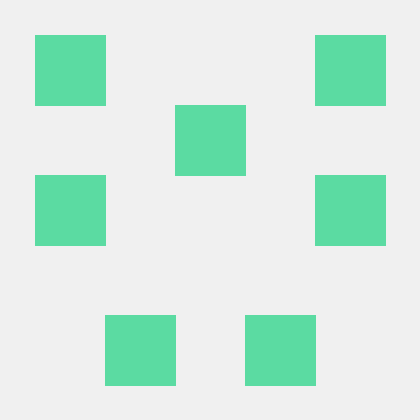
pawanku2/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.- v0.0.0-20190301043612-f6df8288f9b4 go.sum
- v0.0.0-20200121121104-95d072f1b5bb go.sum
Size: 490 MB - Last synced: 18 days ago - Pushed: 20 days ago
