Ecosyste.ms: Repos
An open API service providing repository metadata for many open source software ecosystems.
Package Usage: go: github.com/google/gopacket
Package gopacket provides packet decoding for the Go language.
gopacket contains many sub-packages with additional functionality you may find
useful, including:
Also, if you're looking to dive right into code, see the examples subdirectory
for numerous simple binaries built using gopacket libraries.
Minimum go version required is 1.5 except for pcapgo/EthernetHandle, afpacket,
and bsdbpf which need at least 1.7 due to x/sys/unix dependencies.
gopacket takes in packet data as a []byte and decodes it into a packet with
a non-zero number of "layers". Each layer corresponds to a protocol
within the bytes. Once a packet has been decoded, the layers of the packet
can be requested from the packet.
Packets can be decoded from a number of starting points. Many of our base
types implement Decoder, which allow us to decode packets for which
we don't have full data.
Most of the time, you won't just have a []byte of packet data lying around.
Instead, you'll want to read packets in from somewhere (file, interface, etc)
and process them. To do that, you'll want to build a PacketSource.
First, you'll need to construct an object that implements the PacketDataSource
interface. There are implementations of this interface bundled with gopacket
in the gopacket/pcap and gopacket/pfring subpackages... see their documentation
for more information on their usage. Once you have a PacketDataSource, you can
pass it into NewPacketSource, along with a Decoder of your choice, to create
a PacketSource.
Once you have a PacketSource, you can read packets from it in multiple ways.
See the docs for PacketSource for more details. The easiest method is the
Packets function, which returns a channel, then asynchronously writes new
packets into that channel, closing the channel if the packetSource hits an
end-of-file.
You can change the decoding options of the packetSource by setting fields in
packetSource.DecodeOptions... see the following sections for more details.
gopacket optionally decodes packet data lazily, meaning it
only decodes a packet layer when it needs to handle a function call.
Lazily-decoded packets are not concurrency-safe. Since layers have not all been
decoded, each call to Layer() or Layers() has the potential to mutate the packet
in order to decode the next layer. If a packet is used
in multiple goroutines concurrently, don't use gopacket.Lazy. Then gopacket
will decode the packet fully, and all future function calls won't mutate the
object.
By default, gopacket will copy the slice passed to NewPacket and store the
copy within the packet, so future mutations to the bytes underlying the slice
don't affect the packet and its layers. If you can guarantee that the
underlying slice bytes won't be changed, you can use NoCopy to tell
gopacket.NewPacket, and it'll use the passed-in slice itself.
The fastest method of decoding is to use both Lazy and NoCopy, but note from
the many caveats above that for some implementations either or both may be
dangerous.
During decoding, certain layers are stored in the packet as well-known
layer types. For example, IPv4 and IPv6 are both considered NetworkLayer
layers, while TCP and UDP are both TransportLayer layers. We support 4
layers, corresponding to the 4 layers of the TCP/IP layering scheme (roughly
anagalous to layers 2, 3, 4, and 7 of the OSI model). To access these,
you can use the packet.LinkLayer, packet.NetworkLayer,
packet.TransportLayer, and packet.ApplicationLayer functions. Each of
these functions returns a corresponding interface
(gopacket.{Link,Network,Transport,Application}Layer). The first three
provide methods for getting src/dst addresses for that particular layer,
while the final layer provides a Payload function to get payload data.
This is helpful, for example, to get payloads for all packets regardless
of their underlying data type:
A particularly useful layer is ErrorLayer, which is set whenever there's
an error parsing part of the packet.
Note that we don't return an error from NewPacket because we may have decoded
a number of layers successfully before running into our erroneous layer. You
may still be able to get your Ethernet and IPv4 layers correctly, even if
your TCP layer is malformed.
gopacket has two useful objects, Flow and Endpoint, for communicating in a protocol
independent manner the fact that a packet is coming from A and going to B.
The general layer types LinkLayer, NetworkLayer, and TransportLayer all provide
methods for extracting their flow information, without worrying about the type
of the underlying Layer.
A Flow is a simple object made up of a set of two Endpoints, one source and one
destination. It details the sender and receiver of the Layer of the Packet.
An Endpoint is a hashable representation of a source or destination. For
example, for LayerTypeIPv4, an Endpoint contains the IP address bytes for a v4
IP packet. A Flow can be broken into Endpoints, and Endpoints can be combined
into Flows:
Both Endpoint and Flow objects can be used as map keys, and the equality
operator can compare them, so you can easily group together all packets
based on endpoint criteria:
For load-balancing purposes, both Flow and Endpoint have FastHash() functions,
which provide quick, non-cryptographic hashes of their contents. Of particular
importance is the fact that Flow FastHash() is symmetric: A->B will have the same
hash as B->A. An example usage could be:
This allows us to split up a packet stream while still making sure that each
stream sees all packets for a flow (and its bidirectional opposite).
If your network has some strange encapsulation, you can implement your own
decoder. In this example, we handle Ethernet packets which are encapsulated
in a 4-byte header.
See the docs for Decoder and PacketBuilder for more details on how coding
decoders works, or look at RegisterLayerType and RegisterEndpointType to see how
to add layer/endpoint types to gopacket.
TLDR: DecodingLayerParser takes about 10% of the time as NewPacket to decode
packet data, but only for known packet stacks.
Basic decoding using gopacket.NewPacket or PacketSource.Packets is somewhat slow
due to its need to allocate a new packet and every respective layer. It's very
versatile and can handle all known layer types, but sometimes you really only
care about a specific set of layers regardless, so that versatility is wasted.
DecodingLayerParser avoids memory allocation altogether by decoding packet
layers directly into preallocated objects, which you can then reference to get
the packet's information. A quick example:
The important thing to note here is that the parser is modifying the passed in
layers (eth, ip4, ip6, tcp) instead of allocating new ones, thus greatly
speeding up the decoding process. It's even branching based on layer type...
it'll handle an (eth, ip4, tcp) or (eth, ip6, tcp) stack. However, it won't
handle any other type... since no other decoders were passed in, an (eth, ip4,
udp) stack will stop decoding after ip4, and only pass back [LayerTypeEthernet,
LayerTypeIPv4] through the 'decoded' slice (along with an error saying it can't
decode a UDP packet).
Unfortunately, not all layers can be used by DecodingLayerParser... only those
implementing the DecodingLayer interface are usable. Also, it's possible to
create DecodingLayers that are not themselves Layers... see
layers.IPv6ExtensionSkipper for an example of this.
By default, DecodingLayerParser uses native map to store and search for a layer
to decode. Though being versatile, in some cases this solution may be not so
optimal. For example, if you have only few layers faster operations may be
provided by sparse array indexing or linear array scan.
To accomodate these scenarios, DecodingLayerContainer interface is introduced
along with its implementations: DecodingLayerSparse, DecodingLayerArray and
DecodingLayerMap. You can specify a container implementation to
DecodingLayerParser with SetDecodingLayerContainer method. Example:
To skip one level of indirection (though sacrificing some capabilities) you may
also use DecodingLayerContainer as a decoding tool as it is. In this case you have to
handle unknown layer types and layer panics by yourself. Example:
DecodingLayerSparse is the fastest but most effective when LayerType values
that layers in use can decode are not large because otherwise that would lead
to bigger memory footprint. DecodingLayerArray is very compact and primarily
usable if the number of decoding layers is not big (up to ~10-15, but please do
your own benchmarks). DecodingLayerMap is the most versatile one and used by
DecodingLayerParser by default. Please refer to tests and benchmarks in layers
subpackage to further examine usage examples and performance measurements.
You may also choose to implement your own DecodingLayerContainer if you want to
make use of your own internal packet decoding logic.
As well as offering the ability to decode packet data, gopacket will allow you
to create packets from scratch, as well. A number of gopacket layers implement
the SerializableLayer interface; these layers can be serialized to a []byte in
the following manner:
SerializeTo PREPENDS the given layer onto the SerializeBuffer, and they treat
the current buffer's Bytes() slice as the payload of the serializing layer.
Therefore, you can serialize an entire packet by serializing a set of layers in
reverse order (Payload, then TCP, then IP, then Ethernet, for example). The
SerializeBuffer's SerializeLayers function is a helper that does exactly that.
To generate a (empty and useless, because no fields are set)
Ethernet(IPv4(TCP(Payload))) packet, for example, you can run:
If you use gopacket, you'll almost definitely want to make sure gopacket/layers
is imported, since when imported it sets all the LayerType variables and fills
in a lot of interesting variables/maps (DecodersByLayerName, etc). Therefore,
it's recommended that even if you don't use any layers functions directly, you still import with:
33 versions
Latest release: over 3 years ago
2,918 dependent packages
View more package details: https://packages.ecosyste.ms/registries/proxy.golang.org/packages/github.com/google/gopacket
View more repository details: https://repos.ecosyste.ms/hosts/GitHub/repositories/google%2Fgopacket
Dependent Repos 5,031
sap-contributions/pcap-release Fork of cloudfoundry/pcap-release
A BOSH release and CLI to enable network analysis for CF app developers- v1.1.19 src/pcap/go.mod
- v1.1.19 src/pcap/go.sum
Size: 13.7 MB - Last synced: 16 days ago - Pushed: about 2 months ago

tyler569/hlre
- v1.1.17 router-prototype/go.mod
- v1.1.17 router-prototype/go.sum
Size: 32.2 KB - Last synced: 9 months ago - Pushed: about 1 year ago
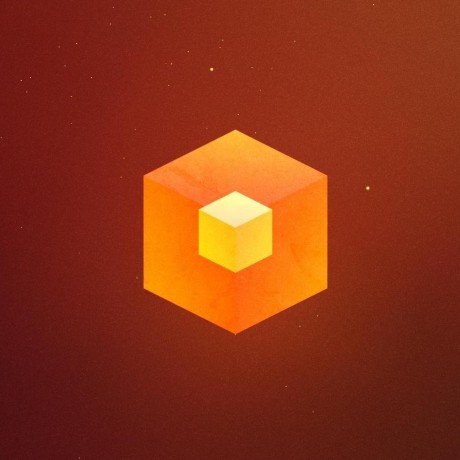
Zondax/rosetta-filecoin-lib
- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 337 KB - Last synced: 10 days ago - Pushed: 11 days ago

drand/drand
🎲 A Distributed Randomness Beacon Daemon - Go implementation- v1.1.19 go.mod
- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 50.9 MB - Last synced: 2 days ago - Pushed: 3 days ago

berty/labs
Berty Labs is a mobile app to explore IPFS on mobile- v1.1.19 go.mod
- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 3.44 MB - Last synced: 1 day ago - Pushed: about 1 year ago
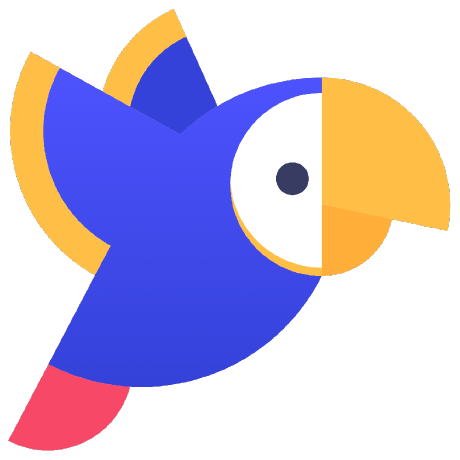
twsnmp/TWLogAIAN
TWSNMP`s Log AI Analyzer- v1.1.17 go.sum
Size: 34.3 MB - Last synced: 2 days ago - Pushed: 10 months ago
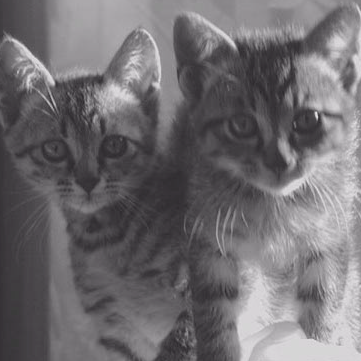
pickkaa/berty Fork of berty/berty
Berty is a secure peer-to-peer messaging app that works with or without internet access, cellular data or trust in the network- v1.1.19 go.mod
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 tool/go.sum
- v1.1.18 tool/go.sum
- v1.1.19 tool/go.sum
Size: 84.2 MB - Last synced: about 7 hours ago - Pushed: about 1 year ago

zaibon/go-ipfs Fork of ipfs/kubo
IPFS implementation in Go- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.17 test/dependencies/go.sum
Size: 40.8 MB - Last synced: about 1 year ago - Pushed: over 1 year ago
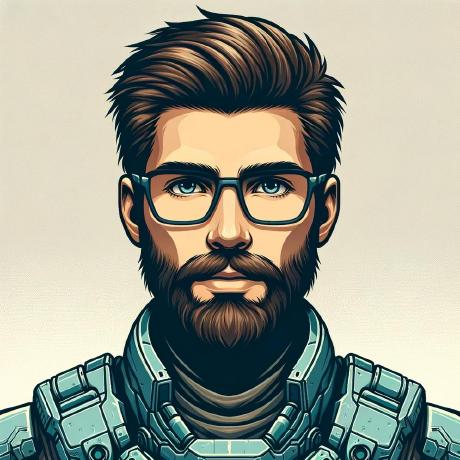
meonBot/go-ipfs Fork of devcode1981/go-ipfs
IPFS implementation in Go- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 test/dependencies/go.sum
Size: 40.8 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

47-studio-org/go-ipfs Fork of MarcelRaschke/go-ipfs
IPFS implementation in Go- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 test/dependencies/go.sum
Size: 46.3 MB - Last synced: 5 months ago - Pushed: 5 months ago

fragmentsh/ssv Fork of bloxapp/ssv
Secret-Shared-Validator(SSV) for ethereum staking- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 19.5 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

filecoin-project/venus-wallet
a remote wallet for provider sign service- v1.1.19 go.mod
- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 1.15 MB - Last synced: 11 days ago - Pushed: 11 days ago

ocfox/nixpkgs Fork of NixOS/nixpkgs
Nix Packages collection- v1.1.14 pkgs/tools/networking/opensnitch/go.mod
- v1.1.14 pkgs/tools/networking/opensnitch/go.sum
Size: 3.57 GB - Last synced: 20 days ago - Pushed: 27 days ago

swimricky/wormhole Fork of wormhole-foundation/wormhole
Certus One's reference implementation for the Wormhole blockchain interoperability protocol.- v1.1.17 event_database/cloud_functions/go.sum
- v1.1.19 event_database/cloud_functions/go.sum
- v1.1.17 event_database/functions_server/go.sum
- v1.1.19 event_database/functions_server/go.sum
- v1.1.19 node/go.mod
- v1.1.17 node/go.sum
- v1.1.19 node/go.sum
Size: 76.7 MB - Last synced: about 1 year ago - Pushed: almost 2 years ago

MatsuriDayo/nekoray
Qt based cross-platform GUI proxy configuration manager (backend: v2ray / sing-box)- v1.1.17 go/go.sum
Size: 2.42 MB - Last synced: 2 months ago - Pushed: 2 months ago

gravwell/training
Gravwell Training Repository- v1.1.17 dockerfiles/go.sum
Size: 38.4 MB - Last synced: 3 days ago - Pushed: 3 days ago

onflow/rosetta
Rosetta implementation for the Flow blockchain- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 453 KB - Last synced: 19 days ago - Pushed: 2 months ago

jmbaur/nixpkgs Fork of NixOS/nixpkgs
Nix Packages collection- v1.1.14 pkgs/tools/networking/opensnitch/go.mod
- v1.1.14 pkgs/tools/networking/opensnitch/go.sum
Size: 3.75 GB - Last synced: about 9 hours ago - Pushed: about 10 hours ago

project-stacker/stacker
Build OCI images natively from a declarative format- v1.1.19 go.sum
Size: 19.1 MB - Last synced: 15 days ago - Pushed: 16 days ago

osinstom/upf-epc Fork of omec-project/upf
4G/5G Mobile Core User Plane- v1.1.19 go.mod
- v1.1.19 go.sum
Size: 5.99 MB - Last synced: 4 days ago - Pushed: 4 days ago

AthanorLabs/atomic-swap
💫 ETH-XMR atomic swap implementation- v1.1.19 go.mod
- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 62.2 MB - Last synced: 10 days ago - Pushed: 5 months ago

jcomeauictx/go-ipfs Fork of ipfs/kubo
IPFS implementation in Go- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 test/dependencies/go.sum
Size: 40.8 MB - Last synced: 3 months ago - Pushed: over 1 year ago

molllyn1/go-ipfs Fork of ipfs/kubo
IPFS implementation in Go- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 test/dependencies/go.sum
Size: 40.9 MB - Last synced: about 1 year ago - Pushed: over 1 year ago

976047167/go-ipfs Fork of ipfs/kubo
IPFS implementation in Go- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.18 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.19 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 test/dependencies/go.sum
- v1.1.17 testplans/bitswap/go.sum
- v1.1.18 testplans/bitswap/go.sum
- v1.1.19 testplans/bitswap/go.sum
Size: 41 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

pradeephubgit/network-measurements Fork of protocol/network-measurements
- v1.1.17 draft-version/geo-asn/go.sum
Size: 28.2 MB - Last synced: about 1 year ago - Pushed: almost 2 years ago

jbarthelmes/go-ipfs Fork of ipfs/kubo 📦
Fork for ipfs/go-ipfs#4224. Built binaries are available: https://github.com/jbarthelmes/go-ipfs/actions?query=workflow%3ABuild+is%3Asuccess- v1.1.17 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.18 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.19 docs/examples/go-ipfs-as-a-library/go.sum
- v1.1.17 go.sum
- v1.1.18 go.sum
- v1.1.19 go.sum
- v1.1.17 test/dependencies/go.sum
- v1.1.17 testplans/bitswap/go.sum
- v1.1.18 testplans/bitswap/go.sum
- v1.1.19 testplans/bitswap/go.sum
Size: 44.4 MB - Last synced: about 1 year ago - Pushed: over 1 year ago

ooni/minivpn
A minimalistic OpenVPN implementation in Go- v1.1.19 go.mod
- v1.1.19 go.sum
Size: 640 KB - Last synced: 20 days ago - Pushed: about 1 month ago

pactus-project/pactus
Pactus blockchain- v1.1.19 go.mod
- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 41.9 MB - Last synced: 19 days ago - Pushed: 19 days ago

rated-network/eth2-crawler Fork of ChainSafe/nodewatch-api
A devp2p crawler targeted at Eth2 nodes- v1.1.17 go.sum
- v1.1.19 go.sum
Size: 6.96 MB - Last synced: about 1 year ago - Pushed: about 1 year ago

vocdoni/vocdoni-node
A set of libraries and tools for the Vocdoni decentralized backend infrastructure, the main ground of our universally verifiable, privacy-centric and scalable digital voting protocol- v1.1.19 go.mod
- v1.1.17 go.sum
Size: 16.3 MB - Last synced: 8 days ago - Pushed: 9 days ago
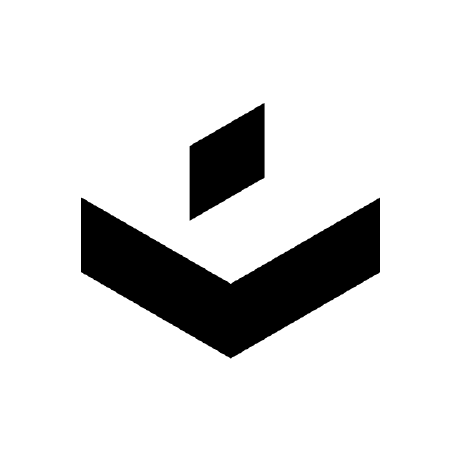