Package Usage: go: github.com/gorilla/sessions
Package sessions provides cookie and filesystem sessions and
infrastructure for custom session backends.
The key features are:
Let's start with an example that shows the sessions API in a nutshell:
First we initialize a session store calling NewCookieStore() and passing a
secret key used to authenticate the session. Inside the handler, we call
store.Get() to retrieve an existing session or a new one. Then we set some
session values in session.Values, which is a map[interface{}]interface{}.
And finally we call session.Save() to save the session in the response.
Note that in production code, we should check for errors when calling
session.Save(r, w), and either display an error message or otherwise handle it.
Save must be called before writing to the response, otherwise the session
cookie will not be sent to the client.
That's all you need to know for the basic usage. Let's take a look at other
options, starting with flash messages.
Flash messages are session values that last until read. The term appeared with
Ruby On Rails a few years back. When we request a flash message, it is removed
from the session. To add a flash, call session.AddFlash(), and to get all
flashes, call session.Flashes(). Here is an example:
Flash messages are useful to set information to be read after a redirection,
like after form submissions.
There may also be cases where you want to store a complex datatype within a
session, such as a struct. Sessions are serialised using the encoding/gob package,
so it is easy to register new datatypes for storage in sessions:
As it's not possible to pass a raw type as a parameter to a function, gob.Register()
relies on us passing it a value of the desired type. In the example above we've passed
it a pointer to a struct and a pointer to a custom type representing a
map[string]interface. (We could have passed non-pointer values if we wished.) This will
then allow us to serialise/deserialise values of those types to and from our sessions.
Note that because session values are stored in a map[string]interface{}, there's
a need to type-assert data when retrieving it. We'll use the Person struct we registered above:
By default, session cookies last for a month. This is probably too long for
some cases, but it is easy to change this and other attributes during
runtime. Sessions can be configured individually or the store can be
configured and then all sessions saved using it will use that configuration.
We access session.Options or store.Options to set a new configuration. The
fields are basically a subset of http.Cookie fields. Let's change the
maximum age of a session to one week:
Sometimes we may want to change authentication and/or encryption keys without
breaking existing sessions. The CookieStore supports key rotation, and to use
it you just need to set multiple authentication and encryption keys, in pairs,
to be tested in order:
New sessions will be saved using the first pair. Old sessions can still be
read because the first pair will fail, and the second will be tested. This
makes it easy to "rotate" secret keys and still be able to validate existing
sessions. Note: for all pairs the encryption key is optional; set it to nil
or omit it and and encryption won't be used.
Multiple sessions can be used in the same request, even with different
session backends. When this happens, calling Save() on each session
individually would be cumbersome, so we have a way to save all sessions
at once: it's sessions.Save(). Here's an example:
This is possible because when we call Get() from a session store, it adds the
session to a common registry. Save() uses it to save all registered sessions.
8 versions
Latest release: 9 months ago
2,897 dependent packages
View more package details: https://packages.ecosyste.ms/registries/proxy.golang.org/packages/github.com/gorilla/sessions
View more repository details: http://repos.ecosyste.ms/hosts/GitHub/repositories/gorilla%2Fsessions
Dependent Repos 20,248
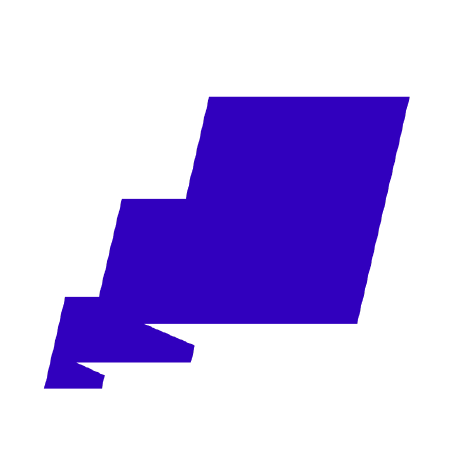

xp-1000/signalfx-agent Fork of signalfx/signalfx-agent
The SignalFx Smart AgentSize: 36.4 MB - Last synced: almost 2 years ago - Pushed: about 2 years ago
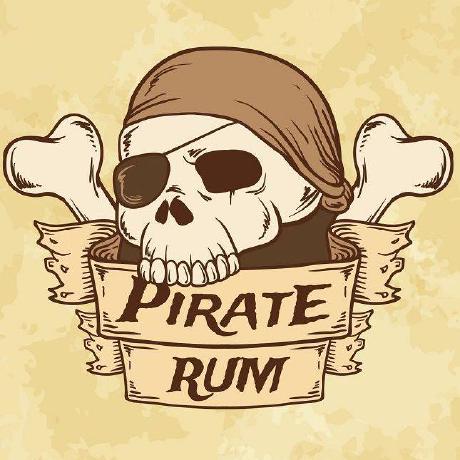
landlordlycat/go-fly Fork of taoshihan1991/go-fly
开源在线客服系统GO语言开发GO-FLY,免费在线客服系统/open source live customer chat by golangSize: 13.7 MB - Last synced: about 1 year ago - Pushed: over 1 year ago

juanmanuel-tirado/terraform-provider-juju Fork of juju/terraform-provider-juju
A Terraform provider for JujuSize: 638 KB - Last synced: almost 2 years ago - Pushed: almost 2 years ago
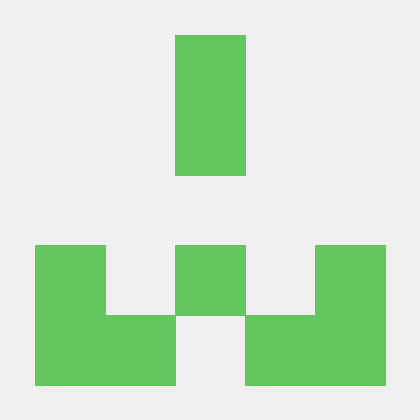
boostchicken/opentelemetry-collector-contrib Fork of open-telemetry/opentelemetry-collector-contrib
Contrib repository for the OpenTelemetry CollectorSize: 262 MB - Last synced: 11 months ago - Pushed: over 1 year ago
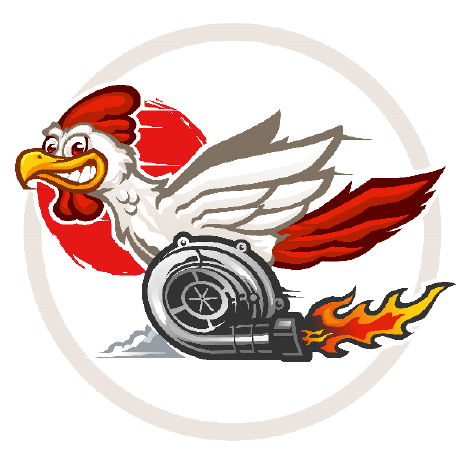
burhankangsi/LetsYouTube
My take on youtube. This app allows upload, download and sharing of content.Size: 884 KB - Last synced: almost 2 years ago - Pushed: almost 3 years ago
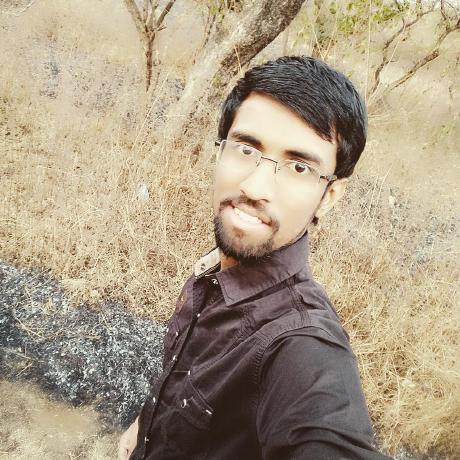
dgfug/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.Size: 420 MB - Last synced: 11 months ago - Pushed: 11 months ago

webability-go/xamboo-env
An ready-to-use environment project for Xamboo ServerSize: 18 MB - Last synced: about 2 months ago - Pushed: about 1 year ago

ali2210/WizDwarf
(:tada:) WzDwarf is a decentralized molecular application interfaceSize: 1.63 GB - Last synced: about 1 month ago - Pushed: about 2 years ago
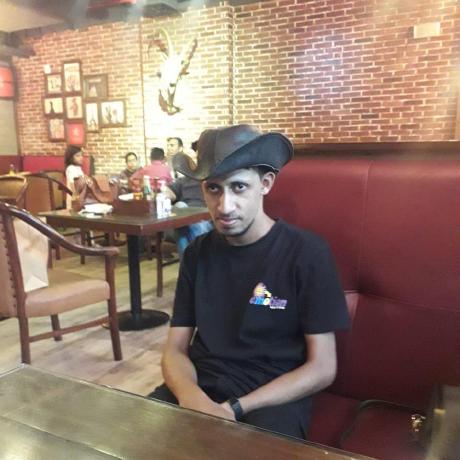
slinkydeveloper/eventing-kafka-broker Fork of knative-sandbox/eventing-kafka-broker
Experimental Kafka Broker implementationSize: 17.4 MB - Last synced: about 2 years ago - Pushed: over 2 years ago

argoproj-labs/argocd-interlace
Enabling Software Supply Chain Security Capabilities in ArgoCDSize: 10.2 MB - Last synced: 2 months ago - Pushed: over 2 years ago

Mu-L/git-lfs Fork of git-lfs/git-lfs
Git extension for versioning large filesSize: 18.6 MB - Last synced: 3 days ago - Pushed: 3 days ago
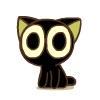
otterize/credentials-operator
Automatically register and generate AWS, GCP & Azure IAM roles, X.509 certificates and username/password pairs for Kubernetes pods using cert-manager, CNCF SPIRE or Otterize CloudSize: 16.6 MB - Last synced: about 8 hours ago - Pushed: 7 days ago

coopstah13/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShiftSize: 446 MB - Last synced: over 1 year ago - Pushed: about 2 years ago

ekmixon/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.Size: 463 MB - Last synced: 11 days ago - Pushed: 11 days ago
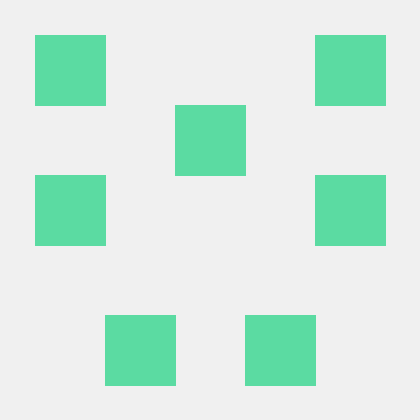
warmchang/nebraska-kinvolk Fork of flatcar/nebraska
Update monitor & manager for applications using the Omaha protocol, optimized for Flatcar Container Linux.Size: 59.2 MB - Last synced: 9 days ago - Pushed: 9 days ago
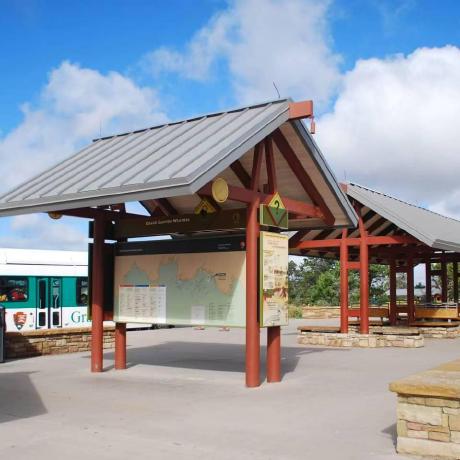
suborbital/reactr
Function scheduler for Go & WebAssemblySize: 556 MB - Last synced: 4 days ago - Pushed: almost 2 years ago

pawanku2/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.Size: 490 MB - Last synced: 6 days ago - Pushed: 6 days ago


francissimo/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.Size: 423 MB - Last synced: about 2 years ago - Pushed: about 2 years ago

soracom/grafana Fork of grafana/grafana
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.Size: 903 MB - Last synced: 6 days ago - Pushed: 7 days ago
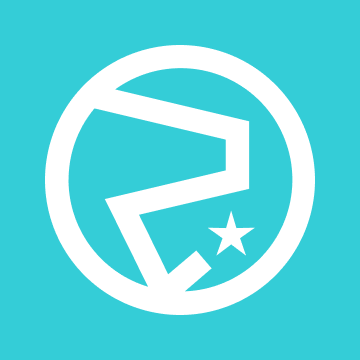
PushkarJ/enhancements Fork of kubernetes/enhancements
Enhancements tracking repo for KubernetesSize: 25.7 MB - Last synced: almost 2 years ago - Pushed: about 2 years ago
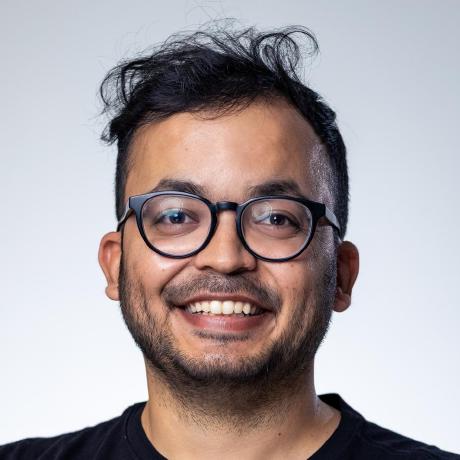
CiscoDevNet/terraform-provider-iosxr
Terraform Cisco IOS-XR ProviderSize: 4.4 MB - Last synced: 6 days ago - Pushed: about 1 month ago

MorningSong/node-problem-detector Fork of kubernetes/node-problem-detector
This is a place for various problem detectors running on the Kubernetes nodes.Size: 27.3 MB - Last synced: 19 days ago - Pushed: 19 days ago
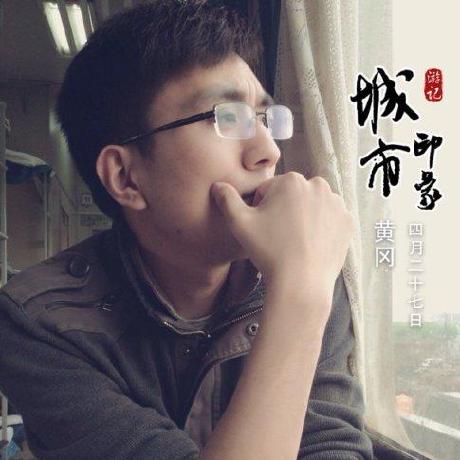
OpenFunction/cli
A CLI for interacting with OpenFunction.Size: 431 KB - Last synced: 11 days ago - Pushed: over 2 years ago

nak3/serverless-operator Fork of openshift-knative/serverless-operator
An aggregation of serverless servicesSize: 65.9 MB - Last synced: over 1 year ago - Pushed: over 1 year ago

Clivern/generator-goapi
🐙 Yeoman Generator for Golang Microservices.Size: 203 KB - Last synced: 6 days ago - Pushed: about 1 month ago

mycrEEpy/mnbcontrol
mnbcontrol is the API & Daemon powering the Midnight Brawlers server landscape.Size: 439 KB - Last synced: about 1 month ago - Pushed: about 1 month ago

flant/negentropy 📦
S - securitySize: 8.88 MB - Last synced: 11 months ago - Pushed: over 2 years ago

nak3/net-kourier Fork of knative-extensions/net-kourier
Purpose-built Knative Ingress implementation using just Envoy with no additional CRDsSize: 40.1 MB - Last synced: over 1 year ago - Pushed: over 1 year ago

xiaojia21190/eagle Fork of go-eagle/eagle
🦅一款小巧的基于Go构建的开发框架,可以快速构建API服务或者Web网站进行业务开发,遵循SOLID设计原则Size: 5.59 MB - Last synced: 22 days ago - Pushed: 22 days ago

dgfug/ethermint Fork of evmos/ethermint
Ethermint is a scalable and interoperable Ethereum, built on Proof-of-Stake with fast-finality using the Cosmos SDK.Size: 30.5 MB - Last synced: 11 months ago - Pushed: about 1 year ago




btrace-baader/kafka-topic-operator 📦
An operator for managing kafka topicsSize: 140 KB - Last synced: 11 months ago - Pushed: almost 3 years ago

rizalgowandy/minio Fork of minio/minio
High Performance, Kubernetes Native Object StorageSize: 94.1 MB - Last synced: 3 months ago - Pushed: 9 months ago

trustbloc/adapter 📦
Integration components to reduce complexity for RPs and IssuersSize: 8.11 MB - Last synced: 11 months ago - Pushed: about 2 years ago

joshiste/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShiftSize: 449 MB - Last synced: 6 months ago - Pushed: over 2 years ago
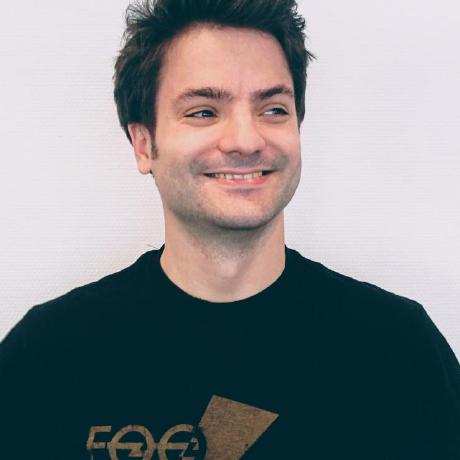
thefreakingmind/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShiftSize: 445 MB - Last synced: about 2 years ago - Pushed: about 2 years ago
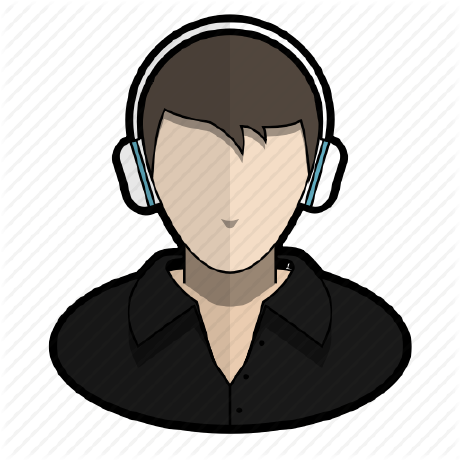
geoand/kubernetes-client Fork of fabric8io/kubernetes-client
Java client for Kubernetes & OpenShiftSize: 447 MB - Last synced: over 1 year ago - Pushed: about 2 years ago
